- FireStart.getControlValue(identifier, onlySelected, delimiter)
- Gets the current value of a form control
- @param {string} identifier - Id of the control
- @param {boolean} onlySelected - If the control is a selection control, this flag determines if all values or only the selected values will be returned. Default is false.
- @param {string} delimiter - Delimiter is used if the value of the control is returned as a CSV, defaults to ';'
- @returns {string} value
- FireStart.setControlValue(identifier, value)
- Sets the current value of a form control
- @param {string} identifier - Id of the control
- @param {string} value - Value the control should have
- FireStart.selectControlValue(identifier, values)
- Selects records of a selection control by an array of IDs
- @param {string} identifier - Id of the Control
- @param {[]} values - Array of values that should be selected
Note: The functions get/set/selectControlValue() operate on the control and return/change the currently displayed control values and also trigger a change to the business entity field if the control is mapped to one.
Example Form
This example shows how to create an HTML control that gets and sets the value of a text field and gets and selects the values of a table using the functions listed above.
The first thing is to design the form and give the controls you would like to use in the HTML control a Title.
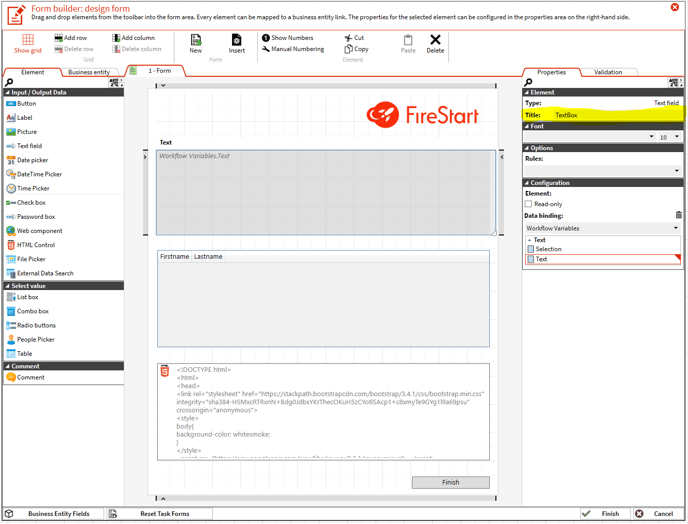
Now you can write as many functions as you like, just make sure to pass the controls as a parameter.
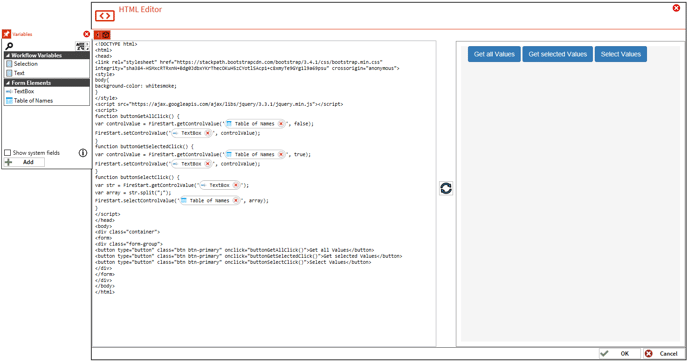
HTML
|
<!DOCTYPE html> |
After you finished and saved your configuration, the workflow is ready to be started.
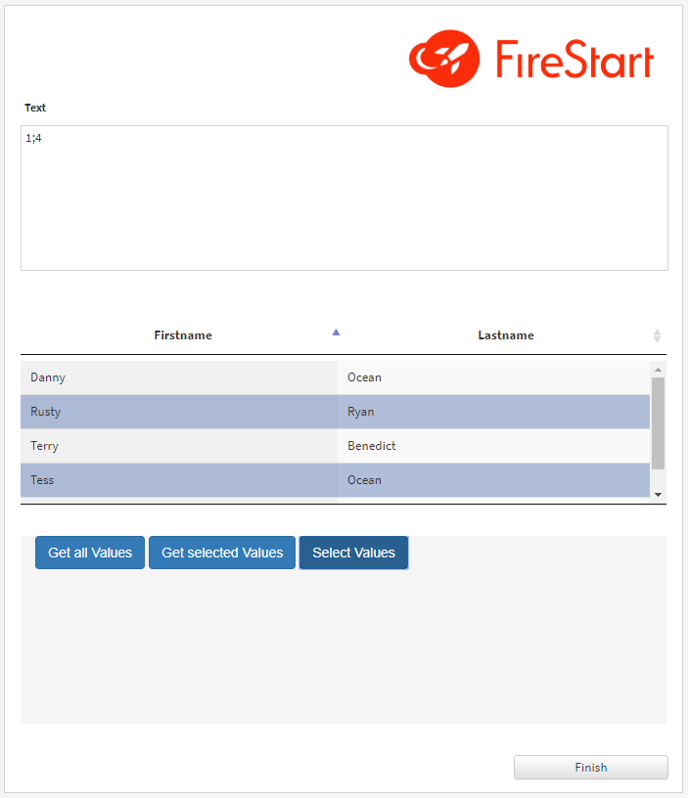